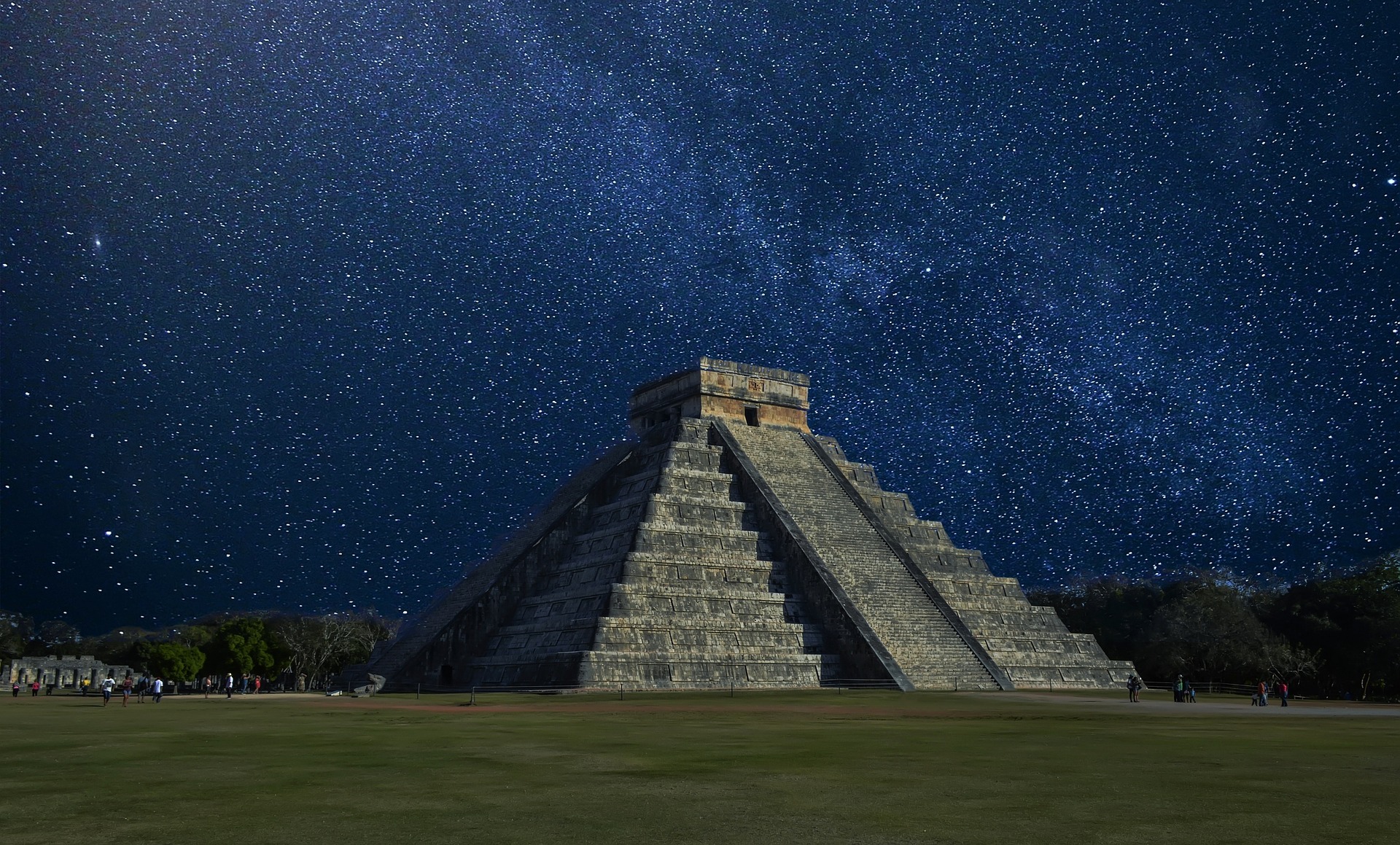
¶一、线程启动、结束,创建线程的多种方法、join,detach
thread
join()
,detach()
,joinable()
- 用类,lambda 表达式创建
1 |
|
¶二、线程传参详解,detach()再探,类成员函数作为线程入口函数
- 传递临时对象作为线程参数,要避免的陷阱
- 临时对象作为线程参数,线程id,临时对象构造时机抓捕
- 传递类对象、只能指针作为线程参数
- 用成员函数指针做线程入口函数
¶2.1 可能的陷阱
传递临时对象作为线程参数
要避免的陷阱
陷阱一:
1 |
|
陷阱二:
1 |
|
总结
- 如果传递简单类型,如内置类型,推荐使用值传递,不要用引用
- 如果传递类对象,避免使用隐式类型转换,全部都是创建线程这一行就创建出临时对象,然后在函数参数里,用引用来接,否则还会创建出一个对象
- 建议不使用 detach
¶2.2 线程 id
线程 Id
通过如下获取:
1 | std::this_thread::get_id(); |
¶2.3 传递 类对象
、 智能指针
作为线程参数
1 |
|
1 |
|
¶三、创建多个进程、数据共享问题分析
¶3.1 创建和等待多个线程
1 | void TextThread() { |
¶3.2 数据共享问题分析
- 只读数据是安全稳定的
- 如果存在写操作,若不加处理就会出错
¶四、互斥量概念、用法、死锁
- 互斥量(mutex)的基本概念
- 互斥量的用法:
lock()
,unlock()
,std::lock_guard
类模板 - 死锁及一般解决方案,
std::lock()
函数模板,std::lock_guard
的std::adopt_lock
参数
¶4.1 互斥量的基本概念及用法
互斥量就是个类对象,可以理解为一把锁,多个线程尝试用 lock()
成员函数来加锁,只有一个线程能锁定成功,如果没有锁成功,那么流程将卡在 lock()
这里不断尝试去锁定。互斥量使用要小心,保护数据不多也不少,少了达不到效果,多了影响效率。
包含 #include<mutex>
头文件,成员函数有, lock()
, unlock()
。基本使用步骤:
lock()
- 操作共享数据
unlock()
lock_guard类模板
1 | lock_guard sbguard(myMutex); // 取代lock() 和 unlock() |
¶4.2 死锁
两个以上互斥量使用,相互竞争,都在争锁,不释放已有锁。主要解决办法是保证多个互斥量上锁的顺序合理。
std::lock()
函数模板
std::lock(mutex1, mutex2,...);
一次性锁定多个互斥量,用于处理多个互斥量。- 如果互斥量中一个没锁住,他就等着,等所有互斥量锁住,才能继续执行。如果有一个没锁住,就会把已经锁住的释放掉(要么互斥量都锁住,要么都没锁住,防止死锁)
**std::lock_guard
** 中 std::adopt_lock
参数
1 | // 加入adopt_lock后,在调用lock_guard的构造函数时,不再进行lock(); |
1 |
|
¶五、unique_lock 模板类
unique_lock
取代lock_guard
(优点:灵活,缺点:效率降低)unique_lock
的第二个参数:std::adopt_lock,std::try_to_lock,std::defer_lock
unique_lock
的成员函数:lock(),unlock(),try_lock(),release()
unique_lock
所有权的传递
¶5.1 unique_lock 的第二个参数:
std::adopt_lock
- 表示这个互斥量已经被
lock()
,即不需要在构造函数中 lock 这个互斥量 - 前提必须提前lock,
lock_guard
也可以用这个参数
std::try_to_lock
- 尝试用 mutex 的
lock()
去锁定这个 mutex,如果没有成功,会立即返回,不会阻塞。 - 使用
try_to_lock()
的原因是:防止其他的线程锁定的 mutex 太长时间,导致本线程一直阻塞在 lock 这个地方 - 前提不能提前 lock()
owns_lock()
方法判断是否拿到锁,如果拿到返回 true
std::defer_lock
- 如果没有第二个参数就对 mutex 加锁,加上
defer_lock
是初始化一个没有加锁的mutex - 不给他加锁的目的是以后可以调用
unique_lock
的一些方法 - 前提不能提前 lock()
unique_lock的成员函数(前三个与 std::defer_lock
联合使用)
lock()
:加锁
1 | unique_lock<mutex> myUniLock(myMutex, defer_lock); // 本质是所有权的转移 |
unlock()
:解锁
1 | unique_lock<mutex> myUniLock(myMutex, defer_lock); |
try_lock()
:尝试给互斥量加锁,如果拿不到返回 false,否则返回 true。
release()
:
1 | unique_lock<mutex> myUniLock(myMutex); // 相当于把myMutex 和 myUniLock绑定在一起,release()就是接触绑定,返回它所管理的mutex对象的指针,并释放所有权 |
注意:
- lock 的代码段越少,执行越快,整个程序的运行效率越高
- 锁住的代码少 -> 细粒度 -> 执行效率高
- 锁住的代码多 -> 粗粒度 -> 执行效率低
unique_lock所有权的传递
- 使用 move 转移
1 | // 只能通过移动语句转移所有权,不能使用拷贝 |
- return 一个临时变量,即可实现转移
1 | unique_lock<mutex> aFuc() { |
¶六、单例设计模式共享数据问题分析、解决,call_once
- 单例设计模式:项目中,有某个或者某些特殊的类,只能创建一个属于该类的对象。
- 单例设计模式共享数据问题分析、解决
std::call_once()
¶6.1 单例模式共享数据问题分析、解决
面临问题:需要在自己创建的线程中来创建单例类的对象,这种线程可能不止一个,可能操作 GetInstance()
这种成员函数需要互斥。
技巧:可以在加锁前判断 m_instance
是否为空,否则每次调用 Singlton::getInstance()
都要进行加锁,十分影响效率。(双重锁定)
1 |
|
如果觉得在单例模式中 new 了一个对象,而没有自己delete ,不合理,可以增加一个类中类 CGarHuiShou
,new 一个单例类时创建一个静态的 CGarhuishou
对象,这样在程序结束时会调用 CGarhuishou
的析构函数,释放掉 new 出来的单例对象。(技巧)
1 | class Singelton { |
¶6.2 std::call_once()
函数模板,该函数的第一个参数为标记,第二个参数是一个函数名(如a())。
功能:能够保证函数a()只被调用一次。具备互斥量的能力,而且比互斥量消耗的资源更少,更高效。call_once()
需要与一个标记结合使用,这个标记为 std::once_flag
;其实 once_flag
是一个结构, call_once()
就是通过标记来决定函数是否执行,调用成功后,就把标记设置为一种已调用状态。
多个线程同时执行时,一个线程会等待另一个线程先执行。
1 | std::once_flag g_flag; |
¶七、condition_variable、wait、notify_one、notify_all
- 条件变量
std::condition_variable
、wait()
、notify_one()
、notify_all
std::condition_variable
实际上是一个类,是一个和条件相关的类,就是等待一个条件成立。
1 | std::mutex mymutex1; |
wait()
用来等一个东西
- 如果第二个参数的lambda表达式返回值是 false,
wait()
将解锁互斥量并阻塞到本行 - 如果第二个参数的lambda表达式返回值是 true,
wait()
直接返回并继续执行
**阻塞到什么时候为止呢?**阻塞到其他某个线程调用 notify_one()
成员函数为止;
如果没有第二个参数,那么效果跟第二个参数lambda表达式返回 false 效果一样 wait()
将解锁互斥量,并阻塞到本行,阻塞到其他某个线程调用 notify_one()
成员函数为止。
当其他线程用 notify_one()
将本线程 wait()
唤醒后,这个 **wait()
**恢复后
1、 wait()
不断尝试获取互斥量锁,如果获取不到,那么流程就卡在 wait()
这里等待获取;如果获取到了,那么 wait()
就继续执行,获取到了锁。
2、如果 wait()
有第二个参数就判断这个 lambda 表达式。
a) 如果表达式为 false,那 wait 又对互斥量解锁,然后又休眠,等待再次被**notify_one()
唤醒
b) 如果lambda表达式为 true,则 wait()
返回,流程可以继续执行(此时互斥量已被锁住**),如果wait没有第二个参数,则 **wait()
**返回,流程走下去。
流程只要走到了 wait()
下面则互斥量一定被锁住了。
1 |
|
深入思考
上面的代码可能导致出现一种情况:
因为 outMsgRecvQueue()
与 inMsgRecvQueue()
并不是一对一执行的,所以当程序循环执行很多次以后,可能在 msgRecvQueue
中已经有了很多消息,但是 outMsgRecvQueue
还是被唤醒一次只处理一条数据。
这时可以考虑把 outMsgRecvQueue
多执行几次,或者对 inMsgRecvQueue
进行限流。
notify_one()
:通知一个线程的 wait()
notify_all()
:通知所有线程的 wait()
¶八、async、future、packaged_task、promise
std::async
、std::future
创建后台任务并返回值std::packaged_task
std::promise
¶8.1 std::async
、std::future
创建后台任务并返回值
需要包含头文件 #include <future>
std::async
是一个函数模板,用来启动一个**异步任务,启动起来一个异步任务之后,它返回一个 std::future
对象,这个对象是个类模板**。
什么叫“启动一个异步任务”?就是自动创建一个线程,并开始执行对应的线程入口函数,返回一个 std::future
对象,这个 std::future
对象中就含有线程入口函数所返回的结果,我们可以通过调用 std::future
对象的成员函数 **get()
**来获取结果。
“future”将来的意思,也有人称呼 std::future
提供了一种访问异步操作结果的机制,就是说这个结果你可能没办法马上拿到,但在不久的将来,这个线程执行完毕时,你就能够拿到结果,所以可以理解为:future中保存着一个值,这个值是在将来的某个时刻能够拿到。
**std::future
**对象的 **get()
成员函数会等待线程执行结束**并返回结果,拿不到结果它就会一直等待,感觉有点像 join()
但是,它是可以获取结果的。
**std::future
**对象的 wait()
成员函数,用于等待线程返回,本身并不返回结果,这个效果和 std::thread
的 join()
更像。
1 |
|
通过向 std::async()
传递一个参数,改参数是 std::launch
类型(枚举类型),来达到一些特殊的目的:
std::lunch::deferred
(defer推迟,延期)
表示线程入口函数的调用会被延迟,一直到 std::future
的 wait()
或者 get()
函数被调用时(由主线程调用)才会执行;如果 wait()
或者 get()
没有被调用,则不会执行。
实际上根本就没有创建新线程。 std::lunch::deferred
意思时延迟调用,并没有创建新线程,是在主线程中调用的线程入口函数。
1 |
|
std::launch::async
,在调用 async 函数的时候就开始创建新线程。
1 | int main() { |
¶8.2 std::async
续谈
std::async
参数详述,async 用来创建一个异步任务
std::launch::deferred
【延迟调用,(主线程调用)】std::launch::async
【强制创建一个线程】
std::async()
一般不叫创建线程(他能够创建线程),一般叫它创建一个异步任务。
std::async
和 std::thread
最明显的**不同,就是 async 有时候并不创建新线程。**
① 如果用std::launch::deferred
来调用 async?
延迟到调用 get()
或者 wait()
时执行,如果不调用就不会执行
② 如果用std::launch::async
来调用 async?
强制这个异步任务在新线程上执行,这意味着,系统必须要创建出新线程来运行入口函数。
③ 如果同时用 std::launch::async | std::launch::deferred
这里这个或者关系意味着 async 的行为可能是 std::launch::async
创建新线程立即执行, 也可能是 std::launch::deferred
没有创建新线程并且延迟到调用 **get()
**执行,由系统根据实际情况来决定采取哪种方案。
④ 不带额外参数 std::async(mythread)
,只给 async 一个入口函数名
此时的系统给的默认值是 std::launch::async | std::launch::deferred
和 ③ 一样,有系统自行决定异步还是同步运行。
std::async
和std::thread()
区别:
std::thread()
如果系统资源紧张可能出现创建线程失败的情况,如果创建线程失败那么程序就可能崩溃,而且不容易拿到函数返回值(不是拿不到)std::async()
创建异步任务。可能创建线程也可能不创建线程,并且容易拿到线程入口函数的返回值;
由于系统资源限制:
① 如果用 std::thread
创建的线程太多,则可能创建失败,系统报告异常,崩溃。
② 如果用 std::async
,一般就不会报异常,因为如果系统资源紧张,无法创建新线程的时候,async 不加额外参数的调用方式就不会创建新线程。而是在后续调用 get()
请求结果时执行在这个调用 get()
的线程上。
如果你强制 async 一定要创建新线程就要使用 std::launch::async
标记。承受的代价是,系统资源紧张时可能崩溃。
③ 根据经验,一个程序中线程数量 不宜超过100~200 。
async 不确定性问题的解决
不加额外参数的 async 调用时让系统自行决定,是否创建新线程。
1 | std::future result = std::async(mythread); |
¶8.3 std::packaged_task:打包任务,把任务包装起来
类模板,它的模板参数是各种可调用对象,通过 packaged_task
把各种可调用对象包装起来,方便将来作为线程入口函数来调用。
1 |
|
¶8.4 std::promise
模板类,能够在某个线程中给它赋值,并可以在其他线程中,把这个值取出来
1 |
|
通过 promise
保存一个值,在将来某个时刻我们通过把一个**future
**绑定到这个 promise
上,来得到绑定的值。注意,使用 thread 时,必须 **join()
**或者 detach()
, 否则程序会报异常。
¶九、future 其他成员函数、shared_future、atomic
std::future
其他成员函数std::shared_future
std::atomic
¶9.1 std::future
的成员函数
1 | std::future_status status = result.wait_for(std::chrono::seconds(5)); |
卡住当前流程,等待 std::async()
的异步任务运行一段时间,然后返回其状态**std::future_status
** 。如果**std::async()
的参数是 std::launch::deferred
(延迟执行),则不会卡住主流程**,没有创建新线程,线程入口函数仍然在遇到 get()
的时候调用,只是主线程中的普通函数调用。std::future_status
是枚举类型,表示异步任务的执行状态。类型的取值有
std::future_status::timeout
std::future_status::ready
std::future_status::deferred
1 |
|
1 |
|
¶9.2 std::shared_future
std::shared_future
:也是个类模板
**
std::future
**的get()
成员函数是转移数据std::shared_future
的get()
成员函数是复制数据
1 |
|
¶9.3 std::atomic
原子操作
有两个线程,对一个变量进行操作,一个线程读这个变量的值,一个线程往这个变量中写值。即使是一个简单变量的读取和写入操作,如果不加锁,也有可能会导致读写值混乱(一条C语句会被拆成3、4条汇编语句来执行,所以仍然有可能混乱);
1 |
|
互斥量:多线程编程中用于保护共享数据:先锁住, 操作共享数据, 解锁。所以通过 mutex 可以解决,但是效率不高。
1 |
|
¶9.4 std::atomic
用法
原子操作:在多线程中不会被打断的程序执行片段。原子操作可以理解成一种:不需要用到互斥量加锁(无锁)技术的多线程并发编程方式。
优势:从效率上来说,原子操作要比互斥量的方式效率要高。
区别:互斥量的加锁一般是针对一个代码段,而原子操作针对的一般都是一个变量。
原子操作,一般都是指“不可分割的操作”;也就是说这种操作状态要么是完成的,要么是没完成的,不可能出现半完成状态。
std::atomic
来代表原子操作,是个类模板,用来封装某个类型的值的。
需要添加头文件 #include <atomic>
。
1 |
|
1 |
|