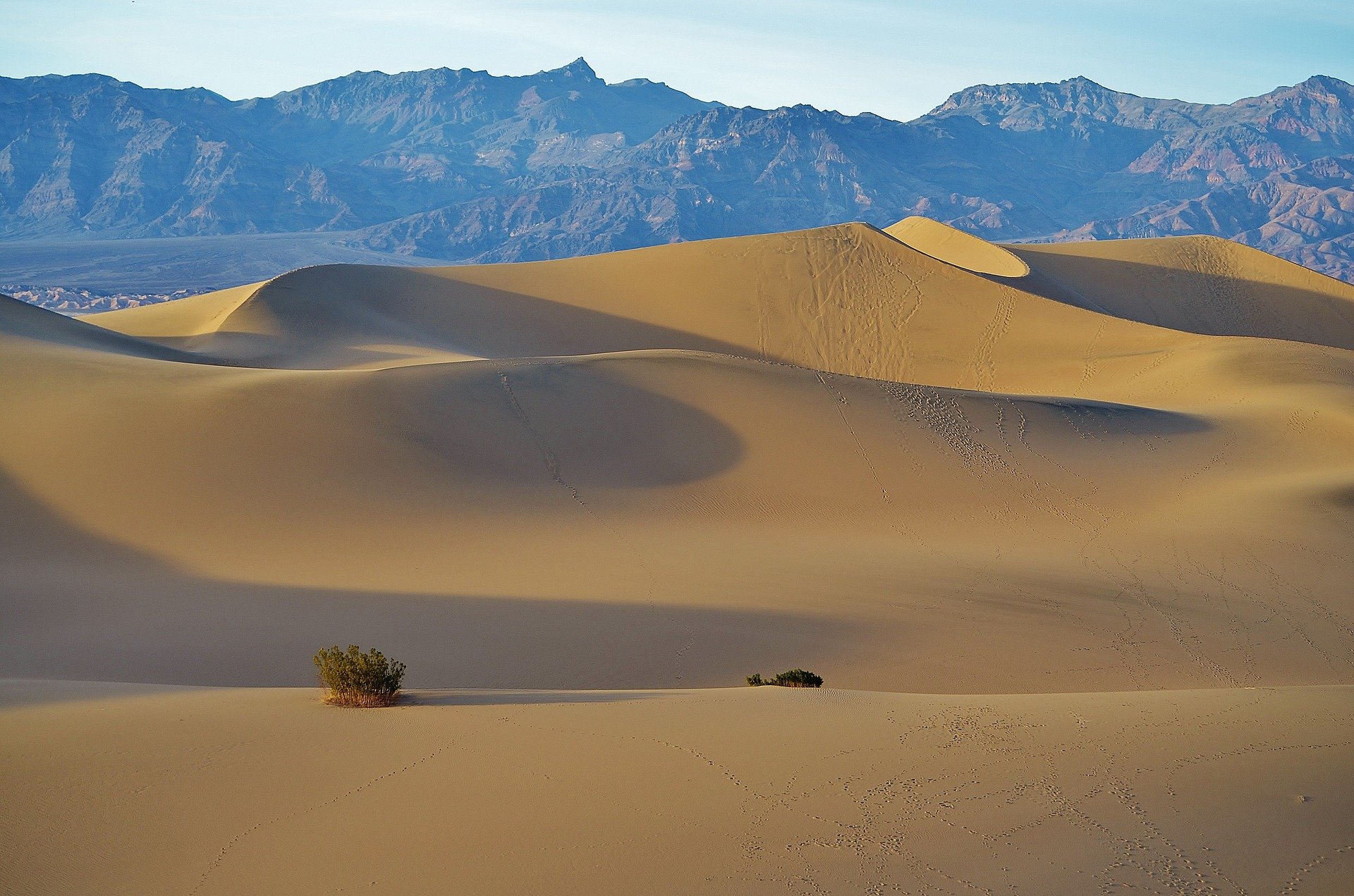
STL_Algorithm
¶0. 文件结构
算法部分总共分为了 5 个部分:
- algo
- algobase
- algoset
- heap
- numeric
¶1. algo
第一部分:
adjacent_find, count, count_if, find, find_if, find_end, find_first_of, for_each, generate, generate_n, remove, remove_copy, remove_if, remove_copy_if, replace, replace_copy,replace_if, replace_copy_if, reverse, reverse_copy, rotate, rotate_copy, search, search_n, swap_range, transform, max_element, min_element, includes, merge, partition, unique, unique_copy
第二部分:
lower_bound, upper_bound, binary_search, next_permutation, prev_permutation, random_shuffle, partial_sort, sort, stable_sort, equal_range, random_shuffle, nth_element, stable_partition
¶1.1 第一部分
直接上例子
1 |
|
《STL源码剖析》 – P338 - P343 (例子出处)
《STL源码剖析》 – P343 - P372 (具体细节)
¶1.2 第二部分
直接上例子:
1 |
|
《STL源码剖析》 – P372 - P374
¶1.2.1 sort
快速排序会为了一些小序列产生大量的递归调用,quick sort
在效率上反而低于 insertion sort
,STL里面的设计了两个 threshold:_lg
, _stl_threshold
。
_lg
用以控制分割恶化的情况,用以选择 quick_sort
或者 heap_sort
;
_stl_threshold
默认为 16 用以作为选择quick_sort
或者 insertion sort
;
1 | // sort 对外接口 |
《STL源码剖析》 – P389 - P400
¶2. algobase
STL 标准规格中并没有区分基本算法或复杂算法,然而SGI却把常用的一些算法定义与<stl_algobase.h>
之中,其他算法定义与 <stl_algo.h>
中,以下一一列举这些所谓的基本算法。以下分为两个部分,第一部分:equal,fill,fill_n,iter_swap,lexicographical_compare,max,min,mismatch,swap
第二部分:copy, copy_backward
¶2.1 第一部分
直接上例子:
1 |
|
《STL源码剖析》 – P306 - P307
¶2.2 第二部分
copy()
的操作脉络如下所示:
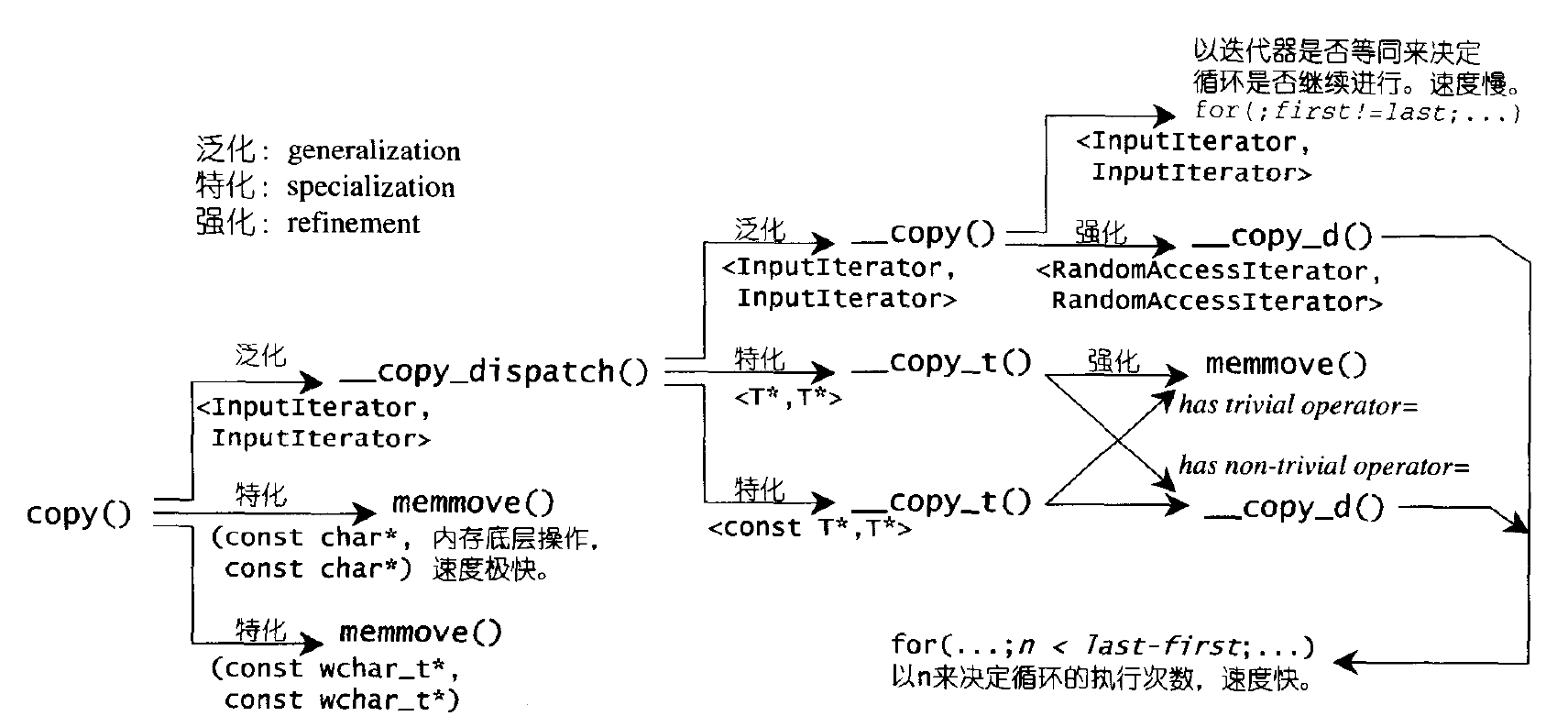
上述的各个版本的的测试见:
《STL源码剖析》 – P321 - P324
**注意:**copy(),以及copy_backward() 需要注意区间重叠的情况。
《STL源码剖析》 – P301, P326
¶3. algoset
STL 一共提供了四种与 set 相关的算法,分别是并集、交集、差集和对称差集。本节的四个算法所接受的 set,必须是有序区间,元素值可以重复出现。
直接上例子:
1 |
|
《STL源码剖析》 – P329 - P330
¶4 heap
包含以下四个函数:make_heap, pop_heap, push_heap, sort_heap
¶5. numeric
该部分的算法,统称为数值算法,欲使用他们必须要包含头文件。
1 |
总共包含了 6 个算法:
accumulate
adjacent_difference
partial_sum
inner_product
power
iota
¶5.1 accumulate
1 | // ~ 累积算法 |
**使用:**详细代码见 5.7
1 | vector<int> iv{2, 3, 4, 5, 6}; |
《STL源码剖析》 – P299 - P300
¶5.2 adjacent_difference
1 | template <class InputIterator, class OutputIterator> // ! 版本一 |
存在两个版本,分别针对默认的邻接差值版本,以及自定义仿函数版本。
使用:
1 | vector<int> iv{2, 3, 4, 5, 6}; |
《STL源码剖析》 – P300 - P301
¶5.3 partial_sum
1 | template <class InputIterator, class OutputIterator> // @ 版本一 |
存在两个版本,分别针对默认累计加法版本,以及自定义仿函数版本。
使用:
1 | vector<int> iv{2, 3, 4, 5, 6}; |
其实 partial_sum
与 adjacent_difference
为互逆运算。
1 | vector<int> iv{2, 3, 4, 5, 6}; |
《STL源码剖析》 – P303 - P304
¶5.4 inner_product
1 | template <class InputIterator1, class InputIterator2, class T> |
存在两个版本,分别针对默认内积版本,以及自定义仿函数版本。
使用:
1 | vector<int> iv{2,3,4,5,6}; |
《STL源码剖析》 – P301 - P302
¶5.5 power
1 | // $ 对x反复执行n次算数操作 |
剑指offer16使用了同样的思想。
使用:
1 | cout << power(10, 3) << endl; |
《STL源码剖析》 – P304 - P305
¶5.6 itoa
1 | // ~ 赋值操作 |
使用:
1 | int n = 3; |
《STL源码剖析》 – P305
¶5.7 综合例子
1 |
|
运行结果如下:
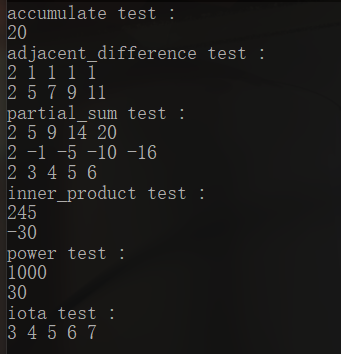
《STL源码剖析》 – P298 - P299
5 参考资料
《STL源码剖析》
MiniSTL / Algorithm 部分