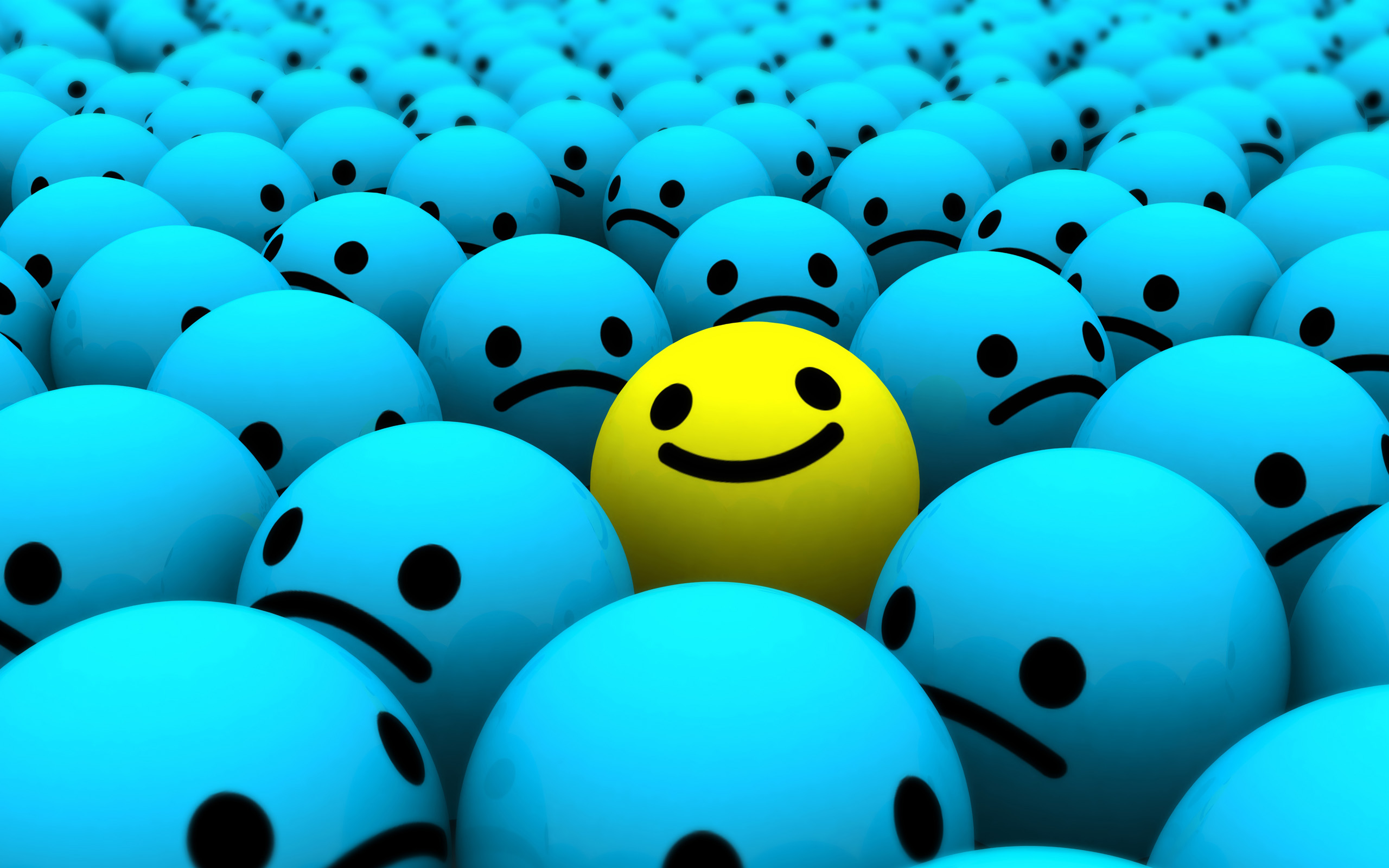
¶剑指offer – 模拟
¶剑指 Offer 29. 顺时针打印矩阵
Difficulty: 简单
输入一个矩阵,按照从外向里以顺时针的顺序依次打印出每一个数字。
示例 1:
1 | 输入:matrix = [[1,2,3],[4,5,6],[7,8,9]] |
示例 2:
1 | 输入:matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]] |
限制:
0 <= matrix.length <= 100
0 <= matrix[i].length <= 100
Solution
1 | class Solution { |
时间复杂度: $O(M\cdot N)$
空间复杂度: $O(1)$,只需要四个方向变量,其中 res 是输出要求空间,不算做算法空间。
¶剑指 Offer 31. 栈的压入、弹出序列
Difficulty: 中等
输入两个整数序列,第一个序列表示栈的压入顺序,请判断第二个序列是否为该栈的弹出顺序。假设压入栈的所有数字均不相等。例如,序列 {1,2,3,4,5} 是某栈的压栈序列,序列 {4,5,3,2,1} 是该压栈序列对应的一个弹出序列,但 {4,3,5,1,2} 就不可能是该压栈序列的弹出序列。
示例 1:
1 | 输入:pushed = [1,2,3,4,5], popped = [4,5,3,2,1] |
示例 2:
1 | 输入:pushed = [1,2,3,4,5], popped = [4,3,5,1,2] |
提示:
0 <= pushed.length == popped.length <= 1000
0 <= pushed[i], popped[i] < 1000
pushed
是popped
的排列。
Solution
1 | class Solution { |
时间复杂度: $O(N)$,同时遍历一次数组
空间复杂度: $O(N)$,额外栈空间
¶参考资料
图解算法数据结构 - LeetBook - 力扣(LeetCode)全球极客挚爱的技术成长平台 (leetcode-cn.com)